Hello, I’m Yuki, a student engineer.
In this article, I would like to explain the requests module as a method of HTTP communication.
What is HTTP communication?
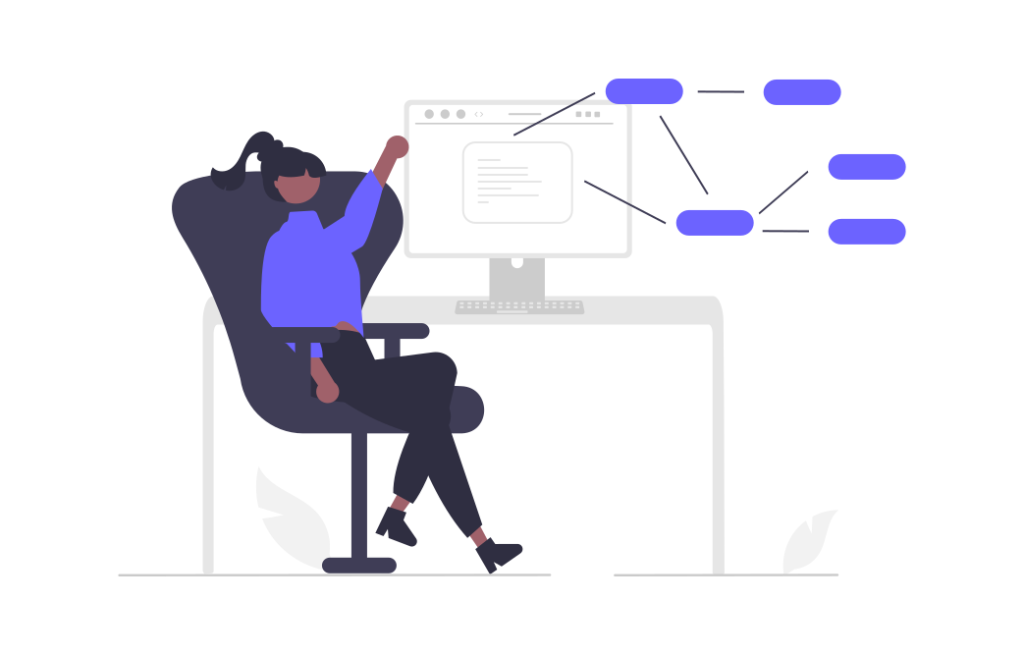
There are four major HTTP methods: GET, POST, PUT, and DELETE.
These four are often used when developing web services and applications using APIs.
In order, they are “data acquisition, creation, update, and deletion.
Now, let’s look at requests, which is an HTTP method in Python.
How to use requests
Installation is performed as follows.
pip install requests
The URL this time is “http://httpbin.org”.
import requests
payload = {'key1': 'value1', 'key2': 'value2'}
#(GET)
r = requests.get('http://httpbin.org/get', params=payload)
#(POST)
r = requests.post('http://httpbin.org/post', data=payload)
#(PUT)
r = requests.put('http://httpbin.org/put', data=payload)
#(DELETE)
r = requests.delete('http://httpbin.org/delete', data=payload)
print(r.status_code)
print(r.text)
print(r.json())
The response of executing get will look like this.
output:
200
{
"args": {
"key1": "value1",
"key2": "value2"
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.24.0",
"X-Amzn-Trace-Id": "Root=1-60729f57-6c4209736ad9a4b805066d50"
},
"origin": "43.234.217.171",
"url": "http://httpbin.org/get?key1=value1&key2=value2"
}
{'args': {'key1': 'value1', 'key2': 'value2'}, 'headers': {'Accept': '*/*', 'Accept-Encoding': 'gzip, deflate', 'Host': 'httpbin.org', 'User-Agent': 'python-requests/2.24.0', 'X-Amzn-Trace-Id': 'Root=1-60729f57-6c4209736ad9a4b805066d50'}, 'origin': '43.234.217.171', 'url': 'http://httpbin.org/get?key1=value1&key2=value2'}
At the end
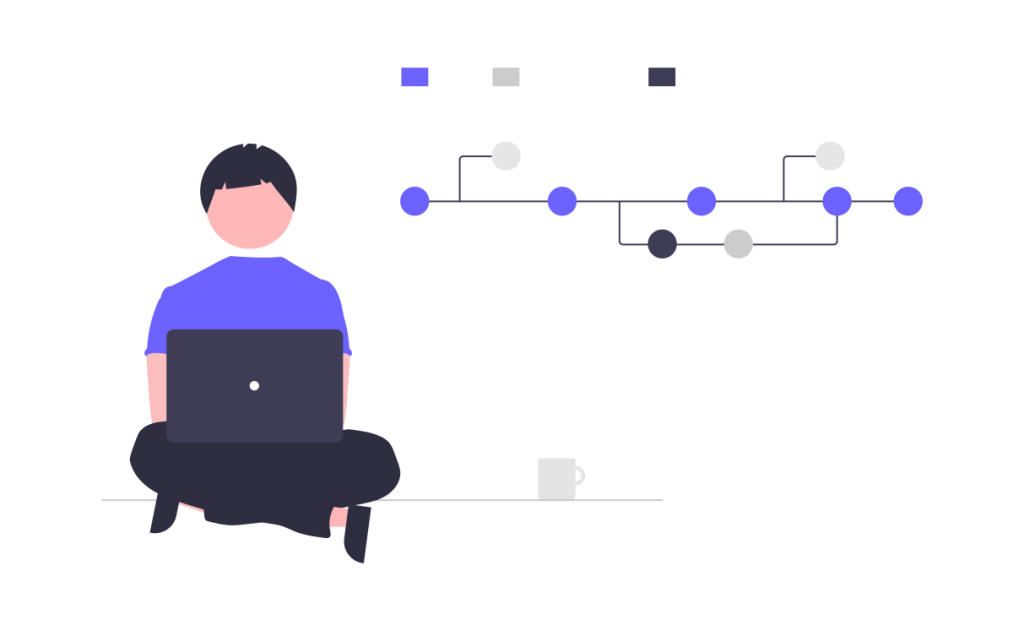
In this article, I explained the requests module as a way to perform HTTP communication.
I think this is a content that is always useful in Python projects.

ゆうき
Thank you for reading!
コメント